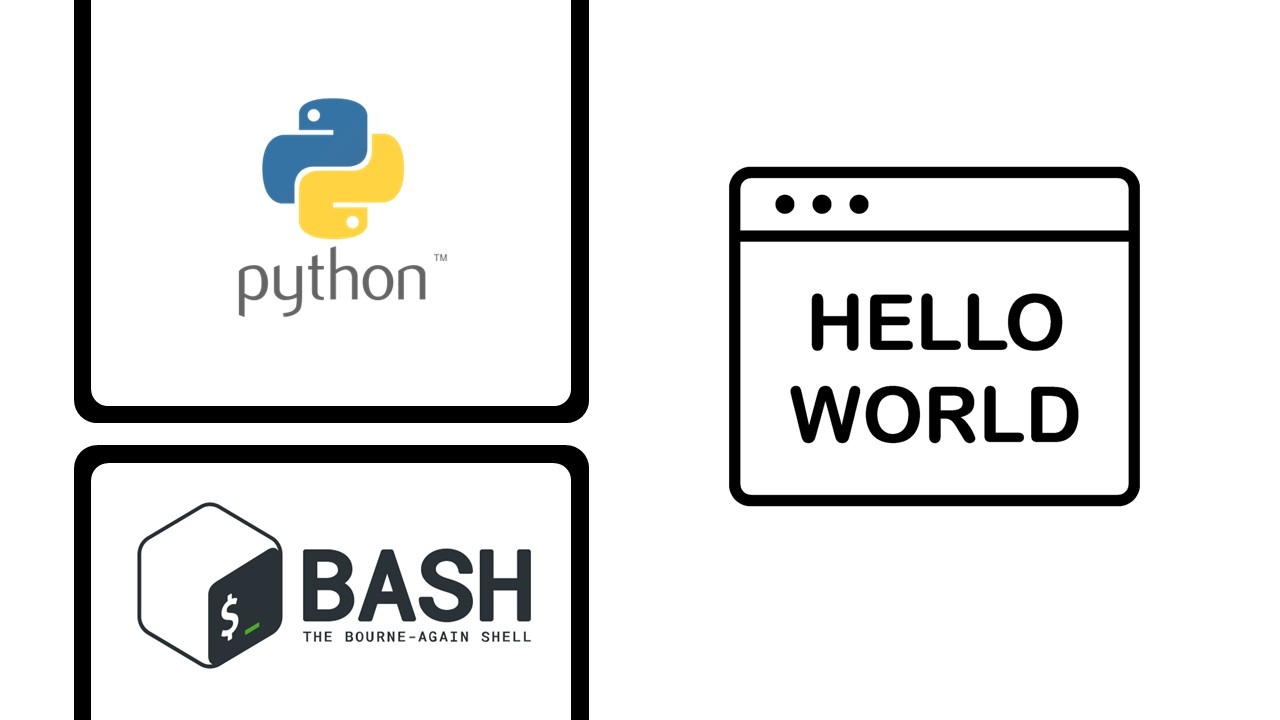
Exploring Programming Shells
In this lab, we'll be exposed to some basic programming concepts while exploring some of the programming shells available in Kali.
BASH
BASH stands for Bourne Again Shell and is a very powerful shell environment that has its own integrated programming or scripting language. A script is just a text file that contains the steps the shell environment will execute to complete a task.
A script is also a series of commands that can be typed at the command prompt but strung together to automate a task. We can write quite complex programs in BASH, but it all begins with a solid knowledge of the basics.
The most basic command in the world of programming is having your code echo "Hello World!" To make this happen in our Kali terminal we simply type in echo "Hellow World!".
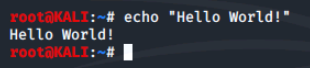
Variables are an important part of programming languages and scripts. A variable is a named placeholder in memory to store a value for later use. This can add flexibility to programs and scripts and is supported by all programming languages.
Let's create a variable called name and store yourname in it.
Note: for this example we will substitute rihanna for yourname.

Notice that no output is returned. If we did not get an error, BASH stored our name in the memory space called name.
Now we'll have the terminal echo our name. We'll use the command echo "Hello $name"
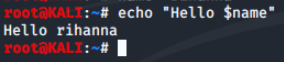
The $ in front of a variable name is how it is referenced for use. This lets BASH know to replace $name with the value stored in the name variable.
While there is a lot we can do from just the prompt, to automate steps we would write a simple script file.
Simple Bash Script
Let's create a simple script to take some user input and then print something back out to the screen. We will first create a blank empty file with the touch command and then edit it with the text editor called nano.
We'll type in the command touch simple-script.sh.
Note: While not required, it is commmon practice to give BASH or shell scripts a .sh file extension.
Once created, we use the nano command to open it.
When we write a script in BASH we need to place what is called the shebang line as the first line. This line lets the shell know which language to run the commands with as the script is processed. Most scripting languages follow this convention.
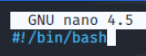
It is good practice to place comments in scripts or programs so future developers will know what the program or specific parts do. In most programming languages a simple one line comment is preceeded by a pound sign or hashtag # and this indicates to the environment that this line is simply a comment and should not be processed.
Next we'll add the lines to request the user enter their name, capture this name and store it in a variable and then print some output to the user.
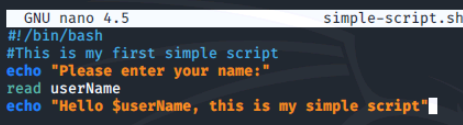
To save in nano press CTRL + O at the same time, click enter to keep the same name, and then CTRL + X to exit nano.
We should be returned to the prompt of the Terminal.
Before we can run our newly created script, we have to give it execute permissions on the Linux system. We will use the chmod 755 simple-script.sh command to do this and then run it with ./simple-script.sh.
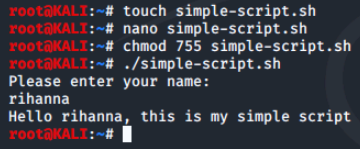
Python
Python is an amazing programming language which can be used to make scripts or full featured programs. We will once again keep things simple in this lab and repeat the steps in Python we did in the previous section. Notice the difference and similarities between BASH and Python. Each language has its own keywords, structure and way of doing things which is called a language's syntax.
We'll type in clear to clear out the contents currently in our terminal. Once complete, we'll type in python.

As with BASH, let's start with a simple Hello World output test. Type print "Hello World!"
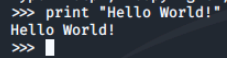
Notice how Python uses print instead of echo which BASH used for output.
Now let's see how variables work in Python. Let's create a variable called name and store yourname in it. Note in this example we will substitute rihanna with yourname.
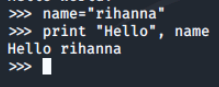
Python does not use the $ in front of a variable name like BASH does. Instead the variable name is directly referenced, but has to be outside of the quotation marks so it is not treated and outputed as a string. Also, we generally should put the content being printed out by the print commmand inside of () characters as we will see if the next section.
Simple Python Script
Let's create a simple script to take some user input and then print something back out to the screen. We will first create a blank empty file with the touch command and then edit it with the text editor called nano.
Using CTRL + D we quit python and return to the terminal prompt. Next, we'll create a file called simple-script.py and edit it in nano.
As with BASH, we should add the shebang line as the first line. This line lets the shell know which language to run the commands with as the script is processed. Python's shebang line is a little different depending upon which version (2 or 3) of Python you wish to use. We will use Python3.
#!/usr/bin/env python3
It is good practice to place comments in scripts or programs so future developers will know what the program or specific parts do. In most programming languages a simple one line comment is preceeded by a pound sign or hashtag # and this indicates to the environment that this line is simply a comment and should not be processed.
Next we will add the lines to request the user enter their name, capture this name and store it in a variable and then print some output to the user. Python lets use do this in one line.
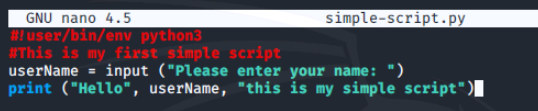
Now, we'll save and exit as we did before. Then we'll use the chmod command to give it execute permissions. Once that's been done, we can run our script. In this case, we will simply use yourname instead of rihanna.
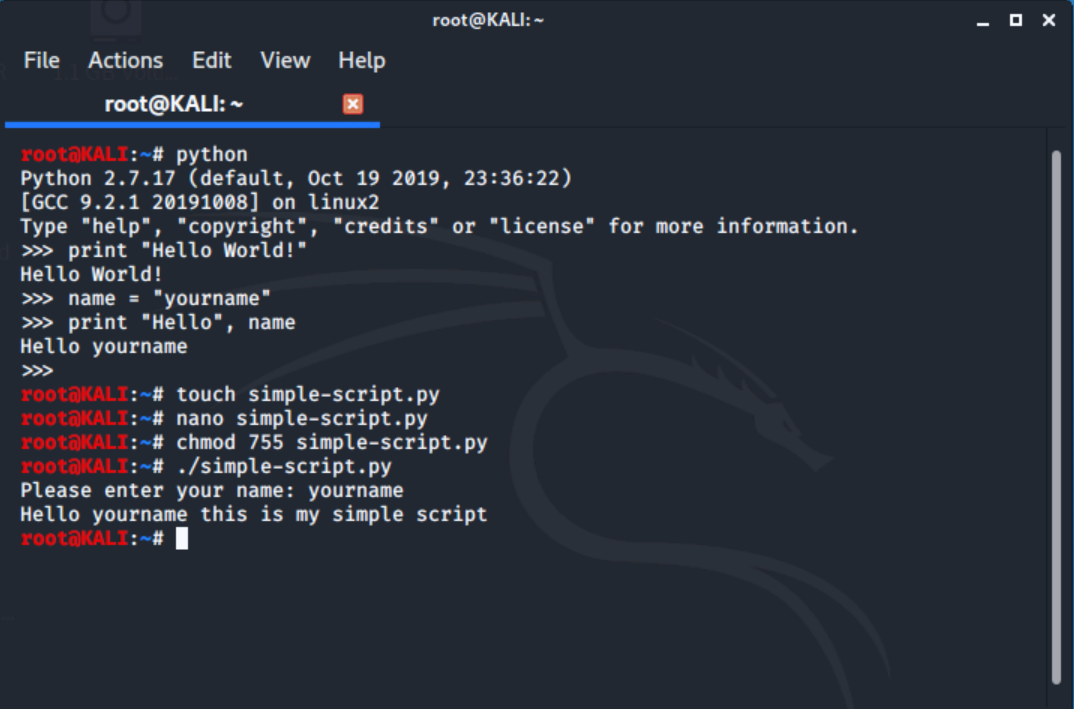
Conclusion
In this lab, we were introduced to programming shells, specifically BASH and Python. We learned that BASH is a powerful shell with its own scripting language, while Python is a versatile programming language.
In BASH, we saw how to write basic programs using commands like "echo" and learned about variables to store values. We also created a simple script to take user input and display output.
Python, on the other hand, uses the "print" statement for output and has its own syntax. We explored variables in Python and created a similar script to capture user input and print output.
0 Comments Add a Comment?