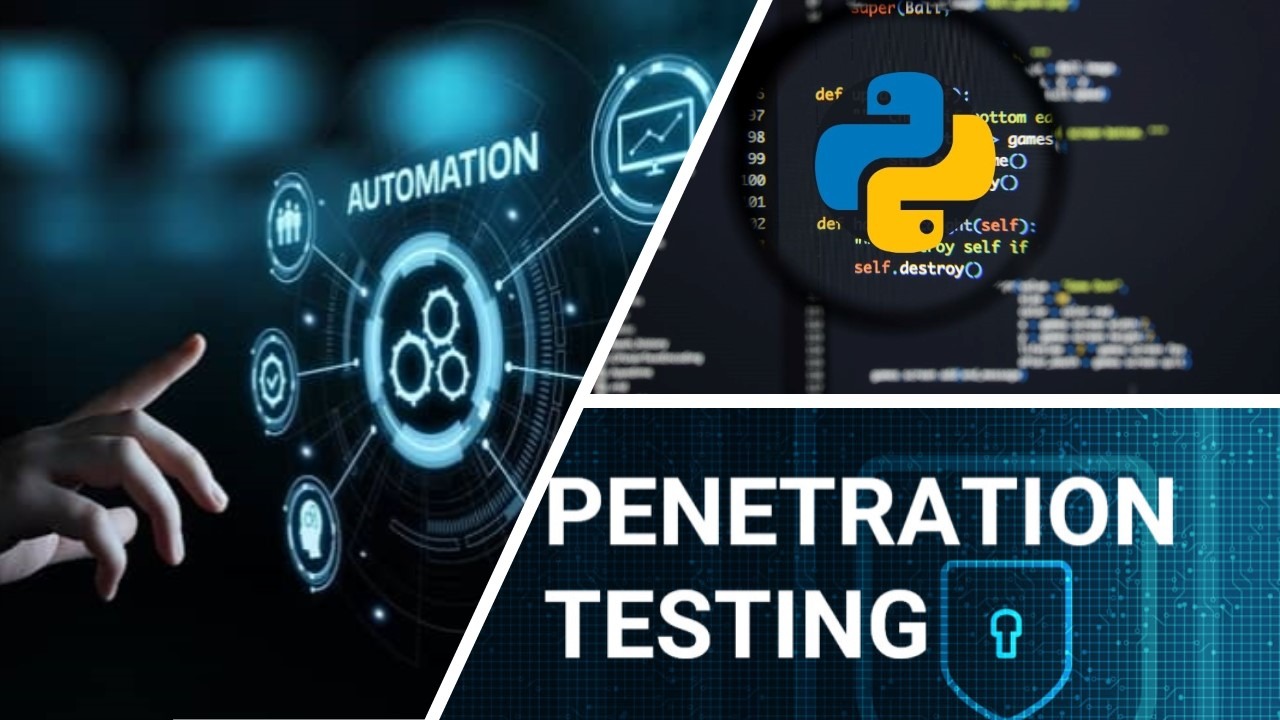
Applying PenTest Automation
In this lab, we'll utilize scripting basics to automate some pentest related tasks.
Automated Reporting in BASH
We are going to create a simple script which will run an nmap scan of Metasploitable2 and then use a program called xsltproc to generate a nice HTML format report.
We'll start off by creating an empty script file called nmap-report.sh and use nano to edit it with the following contents.
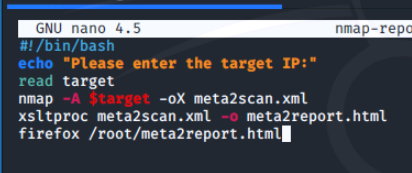
We'll save by using CTRL + O, Press Enter, and then exit nano by using CTRL + X.
We should be returned to the prompt of the Terminal.
Before we can run our newly created script, we have to give it execute permissions on the Linux system. We will use the chmod 755 nmap-report.sh command to do this.
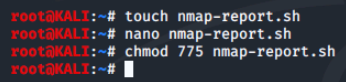
Now we'll execute the script using the command ./nmap-report.sh. The script should ask for the IP of our target. We'll use 10.1.16.9 as our target IP and the script should display the report.

Since we are using the -A option of nmap, it may take a bit to run.
As we can see this can be pretty powerful. There is so much more we could do with the script. For example, we could use the target variable in the naming of the output files so we would not have to hard code the filenames and they would be named based on whatever target we chose.
When the script is complete, we are automatically brought to an Nmap scan report on firefox.
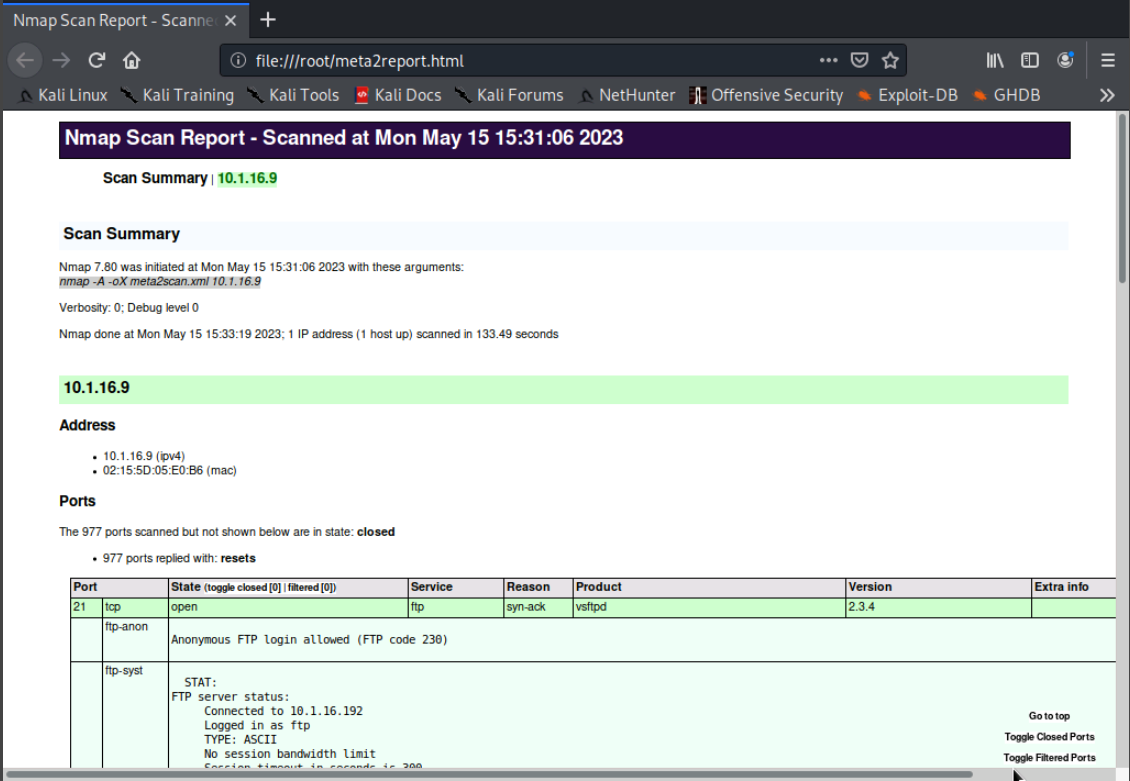
Automated Port Scanner in Python
For this section of the lab, we will create a more complex script in Python which can be used as a port scanner when nmap is not available.
Many penetration testers have a library of such scripts at their disposal for use when they gain access to a device which doesn't have a full load of software (think IoT devices) but still have languages like Python installed. In a relatively few lines of code, this device can be used to pivot and further explore a network.
We'll start by creating a new empty script file called python-scanner.py and insert the following content.
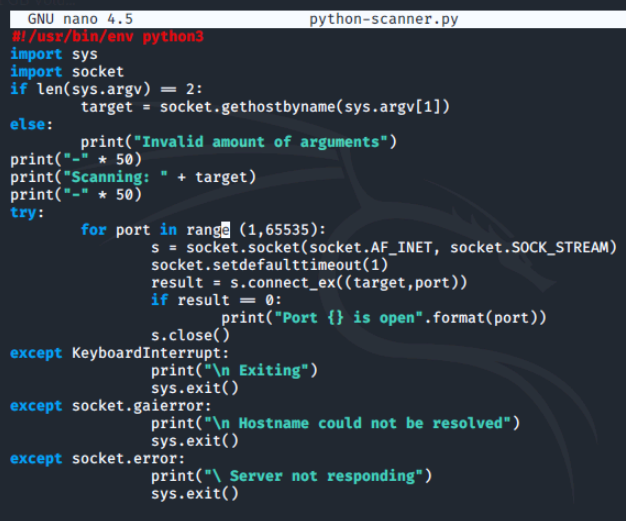
The following lines import the libraries we will be using.
import sys
import socket
This next block of lines sets up how the script will run and get its input. It will take a command line argument of the name or IP of our target and uses an If / Else function to check to ensure a proper argument has been entered on the command line.
if len(sys.argv) == 2:
target = socket.gethostbyname(sys.argv[1])
else:
print("Invalid amount of arguments")
The next block of lines outputs back the target and will show that the script is scanning.
print("-" * 50)
print("Scanning: " + target)
print("-" * 50)
Now the real work of the script begins. Within a try: block, the script will scan all ports from 1 to 65535.
try:
for port in range(1,65535):
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
socket.setdefaulttimeout(1)
The next block uses the returned error code from the socket connection attempt to determine which ports are open and outputs them to the screen.
result = s.connect_ex((target,port))
if result == 0:
print("Port {} is open".format(port))
s.close()
The last blocks of code set up what are called exceptions to deal with any errors encountered during the try block and respond accordingly.
except KeyboardInterrupt:
print("\n Exiting")
sys.exit()
except socket.gaierror:
print("\n Hostname could not be resolved")
sys.exit()
except socket.error:
print("\ Server not responding")
sys.exit()
Now we need to save our script and exit nano.
We should be returned to the prompt of the Terminal.
Before we can run our newly created script, we have to give it execute permissions on the Linux system. We will use the chmod 755 python-scanner.py command to do this.
Then, we run the script using the ./python-scanner.py 10.1.16.9 and display open ports.
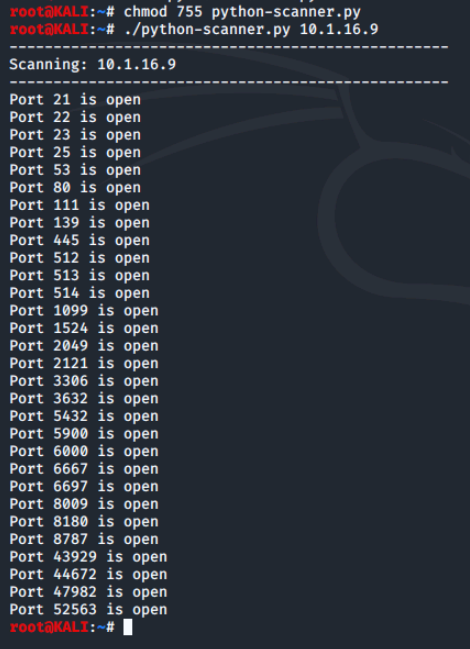
We can see how useful these types of scripts can be for automating various pentest tasks. If we find ourselves typing the same commands at the prompt over and over again to complete a task, it may be time to consider writing a script for it.
Conclusion
In this lab, we learned about the power of automation in penetration testing. We explored two different approaches: automated reporting using BASH and automated port scanning with Python.
In the first part, we created a BASH script that ran an nmap scan on Metasploitable2 and generated a nicely formatted HTML report using xsltproc. By executing the script and providing the target IP, we could automatically generate the report. This showed us how automation can make tasks easier and more efficient.
In the second part, we developed a Python script for port scanning when nmap wasn't available. The script scanned a range of ports and identified open ones. It demonstrated how Python can be used to create simple yet powerful tools for exploring networks and devices.
Overall, these exercises highlighted the benefits of automation in penetration testing. By automating repetitive tasks, we save time, ensure consistency, and improve our testing processes.
0 Comments Add a Comment?